Strings
Introduction
String is a sequences of characters that represent text-based information, such as words, phrases, sentences, or paragraphs. These strings can be composed of any combination of letters, numbers, symbols and other characters. For example, Hello World!
is a string that contains 12 characters.
In this blog post, we’ll dive into PowerShell Strings and explore how they can be used in your applications and scripts.
Declare a String
To declare a string, you can directly assign a string value to a variable and enclose the value with single quote or double quote.
You can also use New-Object
cmdlet. This cmdlet allows you to create objects from .NET classes such as System.String for strings. To create a string object, simply pass the desired string value into the New-Object
cmdlet as an argument:
$str1 = 'Hello World!'
$str2 = "Hello World!"
$str3 = New-Object System.String 'Hello World!'
The above statements will create a new string with the value Hello World!
. You can then access this object like any other variable by referencing its name.
All of below statements will print Hello World!
:
Write-Host $str1
Write-Host $str2
Write-Host $str3
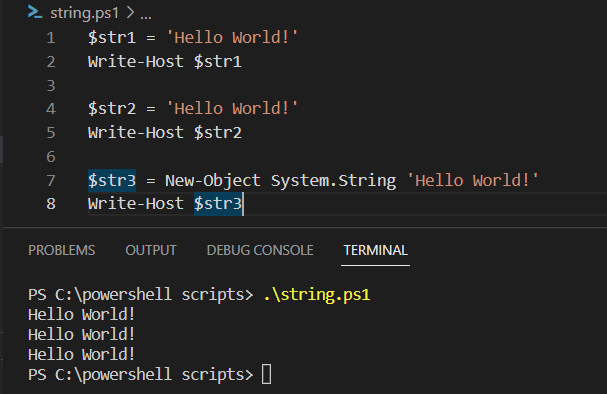
String Indexing
Each string character can be accessed using square bracket []
just like array. The index is zero-based which means the first character is at 0 index.
It can also be accessed using negative index. The last character is at -1 index.
$str1 = 'Hello World!'
Write-Host $str1[4]
Write-Host $str1[-1]
Output:
o
!
Substring
Substring
is used to get range of characters from the string.
There are two overload definitions of Substring
method that can be used.
- taking string from specified index to the rest
- taking string as many as specified length from defined starting index.
Below is the example:
$str1 = 'Hello World!'
Write-Host $str1.Substring(3)
Write-Host $str1.Substring(3,5)
Output:
lo World!
lo Wo
To get list of overload methods from a given method, you can invoke the name of method from the object as below:
"a".Substring
"a".Replace
Output:
OverloadDefinitions
-------------------
string Substring(int startIndex)
string Substring(int startIndex, int length)
string Replace(string oldValue, string newValue, bool ignoreCase, cultureinfo culture)
string Replace(string oldValue, string newValue, System.StringComparison comparisonType)
string Replace(char oldChar, char newChar)
string Replace(string oldValue, string newValue)
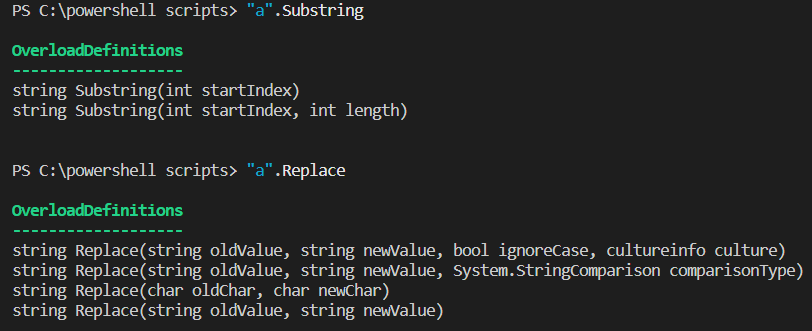
Split
Split
is used to split string based on separators.
Below example will split string based on comma:
$str = "a,b,c,d,e"
$str.Split(',')
Output:
a
b
c
d
e
Based on overload definitions of Split
method, you can add option to remove blank value.
$str = "a,,c,d,e"
$str.Split(',', [StringSplitOptions]::RemoveEmptyEntries)
Output:
a
c
d
e
Since Split
method returns array, you can deconstruct the return value.
$str = "a,b,c"
$a, $b, $c = $str.Split(',')
$a
$b
$c
Output:
a
b
c
Replace
Replace
method is used to replace string with a new string or character.
Below example will replace part of the string with new value.
$str = "John Doe"
$str.Replace("Doe","Lennon")
Output:
John Lennon
Below example will replace part of the string with new character.
$str = "John Doe"
$str.Replace("o","u")
Output:
Juhn Due
Check whether a variable is a string or not
To check wheter a variable is a string or not, you can invoke GetType
method.
$str1 = 'Hello World!'
$str1.GetType()
Output:
IsPublic IsSerial Name BaseType
-------- -------- ---- --------
True True String System.Object
Conclusion
String is incredibly useful for creating and manipulating text-based data within your applications and scripts. Whether it’s storing messages or generating dynamic content on webpages, working with strings is an essential part of any programmer’s toolkit!
With that said though, it’s important to remember that there are many more powerful features available within PowerShell, so make sure to check out Microsoft’s official documentation if you want to learn more about what else it has to offer!