How to Combine Multiple Paths in PowerShell
Problem
In this blog post, we will show you how to combine multiple paths in PowerShell.
Using Join-Path Cmdlet
You can join paths using Join-Path
cmdlet. This cmdlet allows you to combine multiple path segments into a single, well-formed path.
This method is the most recommended way since it handles the path separators correctly and ensures cross-platform compatibility.
The example is as follows:
# Define your path segments
$folderPath = "C:\Users"
$folderName = "Michael"
$fileName = "example.txt"
# Join the path segments
$fullPath = Join-Path -Path $folderPath -ChildPath $folderName
$fullPath = Join-Path -Path $fullPath -ChildPath $fileName
# Display the result
Write-Host "$fullPath"
In this example:
- We define the individual path segments, such as
$folderPath
,$folderName
and$fileName
- We use
Join-Path
cmdlet to combine these segments into a single path. We start by joining$folderPath
and$folderName
, and then we join the result with$fileName
. - Finally, we display the full path using
Write-Host
.
The output will be C:\Users\Michael\example.txt
.
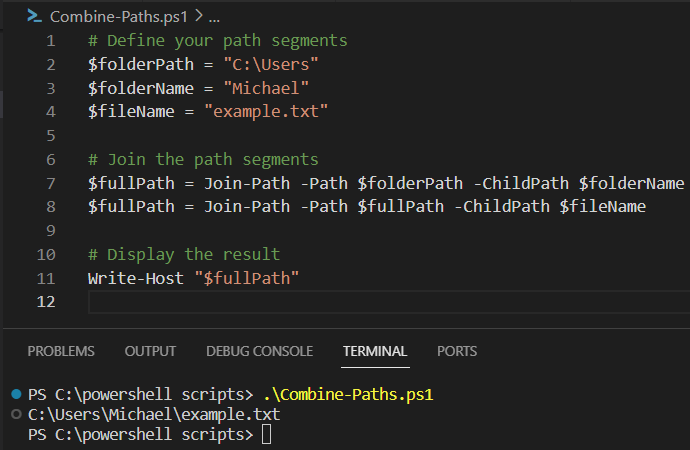
Using .NET Framework Path.Combine Method
Since .NET Framework is deeply integrated with PowerShell, you can always resort to built-in library provided by .NET Framework, such as Path.Combine
static method that can be used to combine multiple paths.
# Define your path segments
$folderPath = "C:\Users"
$folderName = "Michael"
$fileName = "example.txt"
# Use .NET Path.Combine method to create the path
$fullPath = [System.IO.Path]::Combine($folderPath, $folderName, $fileName)
# Display the result
Write-Host "$fullPath"
The output will be the same as before which is C:\Users\Michael\example.txt
and this is the second most reliable way to combine paths in PowerShell.
Using String Concatenation
You can concatenate path segments using the +
operator.
# Define your path segments
$folderPath = "C:\Users"
$folderName = "Michael"
$fileName = "example.txt"
# Join the path segments using string concatenation
$fullPath = $folderPath + "\" + $folderName + "\" + $fileName
# Display the result
Write-Host "$fullPath"
The output will be the same as before, i.e., C:\Users\Michael\example.txt
.
Using String Format
You can also use string formatting to create a path by inserting the path segments into a format string.
# Define your path segments
$folderPath = "C:\Users"
$folderName = "Michael"
$fileName = "example.txt"
# Use string formatting to create the path
$fullPath = "{0}\{1}\{2}" -f $folderPath, $folderName, $fileName
# Display the result
Write-Host "$fullPath"
The output will be C:\Users\Michael\example.txt
as before.
Using String Interpolation
PowerShell supports string interpolation which allows you to embed variables directly into double-quoted strings. You can use this feature to join paths.
# Define your path segments
$folderPath = "C:\Users"
$folderName = "Michael"
$fileName = "example.txt"
# Use string interpolation to create the path
$fullPath = "$folderPath\$folderName\$fileName"
# Display the result
Write-Host "$fullPath"
Using String Joining Operator
PowerShell provides the -join
operator which you can use to concatenate elements of an array with a specified separator. You can use this operator to join path segments.
# Define your path segments
$folderPath = "C:\Users"
$folderName = "Michael"
$fileName = "example.txt"
# Define your path segments as an array
$pathSegments = @($folderPath, $folderName, $fileName)
# Join the path segments using the -join operator
$fullPath = $pathSegments -join "\"
# Display the result
Write-Host "$fullPath"
Conclusion
There are many methods that can be used to combine multiple paths in PowerShell. The most reliable methods are using Join-Path
cmdlet and .NET Framework Path.Combine
Method since it ensures cross-platform compatibility.
The other methods can still be used particularly in specific operating system and for simple cases.