How to Pad a Number with Leading Zeros in PowerShell
Problem
In this article, I will show you how to pad a number with leading zeros in PowerShell.
Using ToString Method
You can use the ToString
method with a format specifier to pad zeros before an integer.
In this example, the D2
format specifier is used to ensure that the integer is always displayed with at least two digits, and leading zeros are added if necessary. If the number is already two digits or more, no padding is added.
for ($i = 0; $i -le 10; $i++) {
$paddedNumber = $i.ToString("D2")
Write-Host $paddedNumber
}
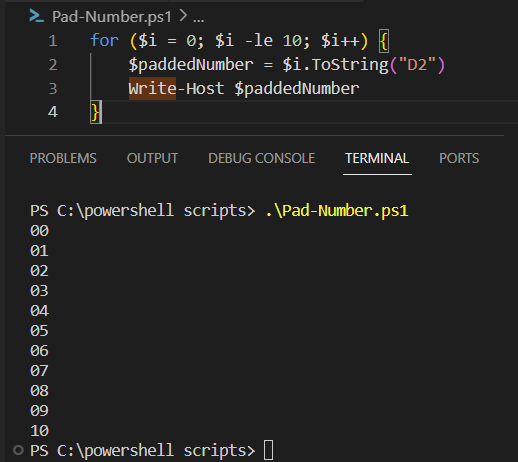
This method is derived from C#
method in .NET Framework since PowerShell is built on .NET Framework.
Using PowerShell Format Operator
You can also use PowerShell format operator, i.e., -f
to format the string. Additionally, {0:D2}
is the format specifier that ensures a minimum width of 2 digits with leading zeros.
for ($i = 0; $i -le 10; $i++) {
$paddedNumber = "{0:D2}" -f $i
Write-Host $paddedNumber
}
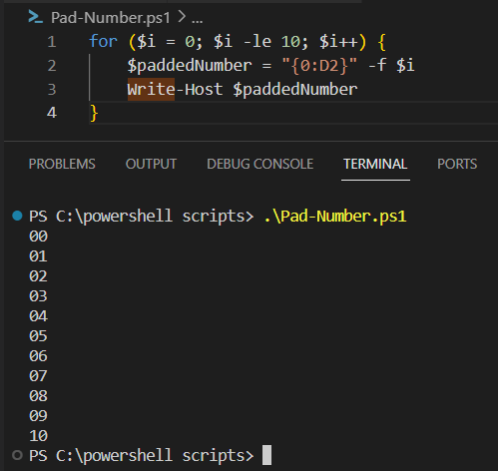
Using String Format Method
This one is another solution to achieve the same result. This method is also derived from C# String Formatting method in .NET Framework. The {0:D2}
format specifier within String.Format
method will zero-pad the number.
for ($i = 0; $i -le 10; $i++) {
$paddedNumber = [string]::Format("{0:D2}", $i)
Write-Host $paddedNumber
}
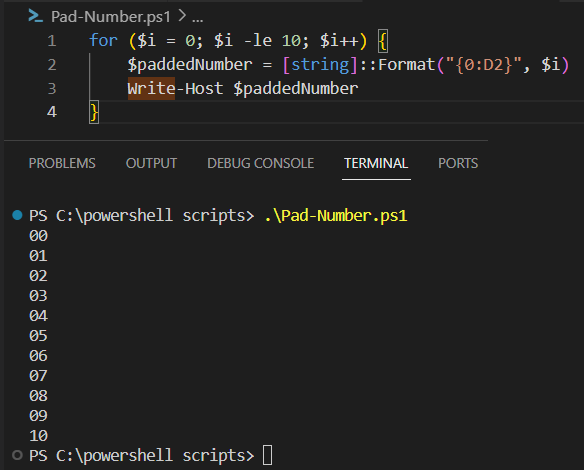
Using PadLeft Method
PadLeft
is another method from C# that can be used to pad a number with leading zeros.
In this solution, ToString()
method is used to convert the integer to a string, and then PadLeft(2, '0')
is applied to ensure that the string representation has a minimum width of 2 characters with leading zeros.
for ($i = 0; $i -le 10; $i++) {
$paddedNumber = $i.ToString().PadLeft(2, '0');
Write-Host $paddedNumber
}
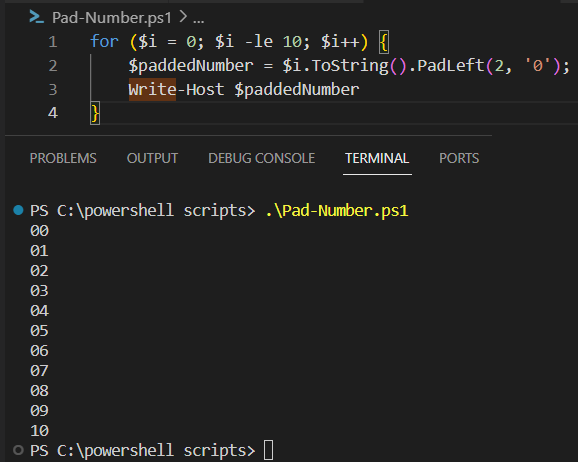
Conclusion
There are many approaches that can be used to pad a number with leading zeros. We can use ToString
method with format specifier. We can also use string Format
and PadLeft
methods. Besides that, PowerShell also has format operator, i.e., -f
, that can achieve the same result.