How to Check Leap Year in PowerShell
Introduction
In this blog post, we will walk through how to check leap year in PowerShell.
Solution
Using System.DateTime Class from .NET Framework
We can use static method from System.DateTime
class to check leap year as in following example:
$year = 2024
[System.DateTime]::IsLeapYear($year)
The output of above script is true
because 2024 is a leap year. But, if we change the year to 2018, the output will be false
.
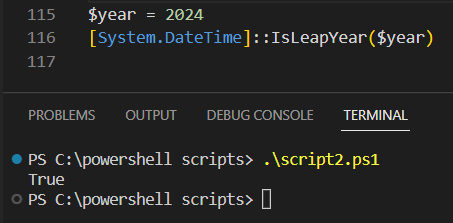
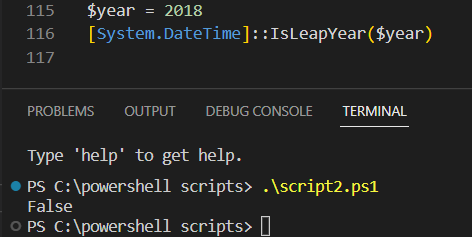
Using arithmetic and logical operator
By definition, a leap year must be divisible by 4 unless the year is divisible by 100 as well but not by 400. So, we can create simple algorithm combining arithmetic and logical operator to check leap year as follows:
$year = 4000
$isLeapYear = $year % 4 -eq 0 -and ($year % 100 -ne 0 -or $year % 400 -eq 0)
Write-Host $isLeapYear
The result of above script is true. However, if the year is changed to 4100, the result will be false because even though 4100 is divisible by 4 and 100, it’s not divisible by 400.
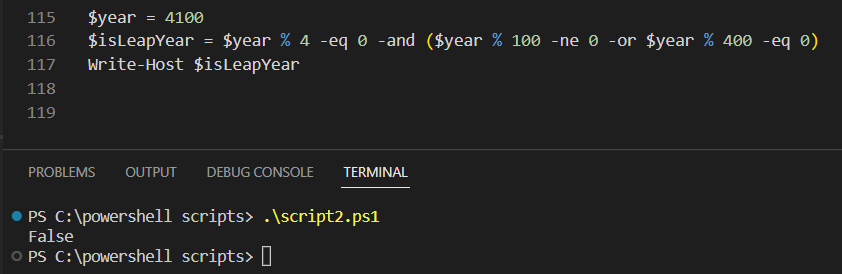
Conclusion
In conclusion, to check leap year in PowerShell we can use IsLeapYear
static method from .NET Framework. Besides that, we can also implement simple algorithm from scratch to check leap year.