How to Measure Execution Time of PowerShell Script
Problem
Measuring PowerShell script execution time is important when we have different implementations of a script for the same task and we want to find the most efficient one.
In this blog post, we will show you how to measure execution time of PowerShell script.
Using Measure-Command Cmdlet
You can measure the execution time of a script or a specific part of the code using Measure-Command
cmdlet.
Measure-Command { Get-AppxPackage }
Suppose you have PowerShell script file named my-script.ps1
and want to measure its execution time, you can put the file inside Measure-Command
script block as follows:
Measure-Command { .\my-script.ps1 }
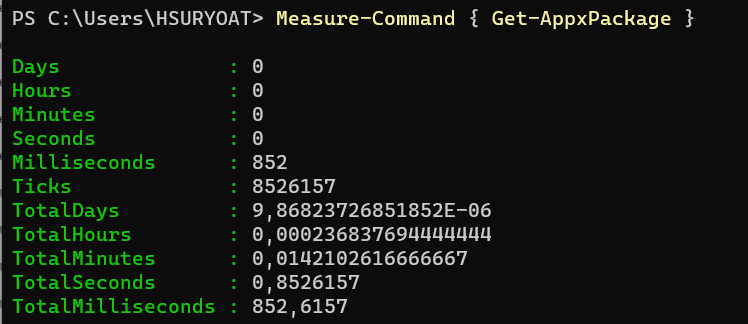
After running Measure-Command
cmdlet, PowerShell will display the output which includes properties like Days, Hours, Minutes, Seconds, and Milliseconds. These properties provide the execution time of the script in different units.
Using Get-Date Cmdlet
You can record the start and end time of the script execution using Get-Date
cmdlet and then calculate the time difference.
$startTime = Get-Date
# Your script code goes here
Get-AppxPackage # Simulate some task taking time
$endTime = Get-Date
$executionTime = $endTime - $startTime
Write-Host "Script execution time: $executionTime"
The result will look as follows:
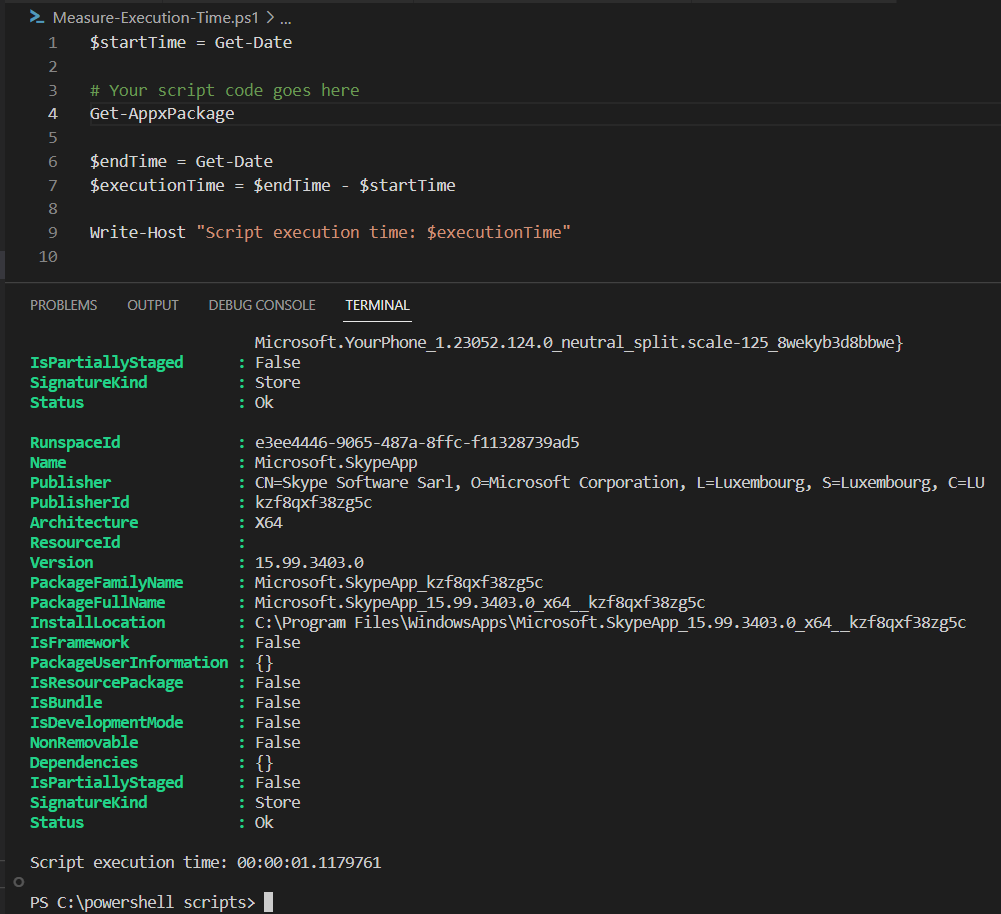
Using Stopwatch Class
Since PowerShell is deeply integrated to .NET Framework, we can use Stopwatch
class from System.Diagnostics.Stopwatch
namespace.
$stopwatch = [System.Diagnostics.Stopwatch]::StartNew()
# Your script code goes here
Get-AppxPackage
$stopwatch.Stop()
$executionTime = $stopwatch.Elapsed
Write-Host "Script execution time: $executionTime"
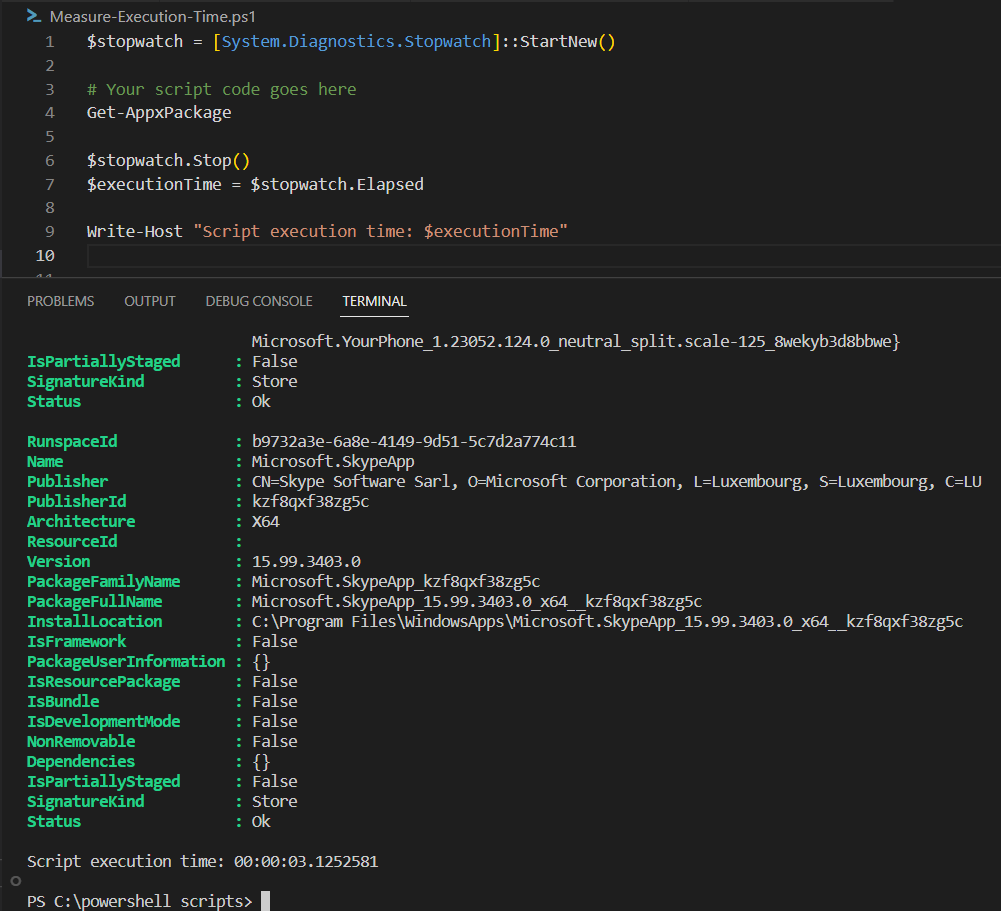
Using Start-Transcript and Stop-Transcript Cmdlets
These cmdlets are actually used to record all PowerShell activities in one session. It will capture the entire script’s output, including the execution time, into a log file.
Start-Transcript -Path "ScriptLog.txt"
# Your script code goes here
Get-AppxPackage
Stop-Transcript
$start = ''
Get-Content -Path "ScriptLog.txt" | Select-String "Start time"
| ForEach-Object {
$start = ($_.Line ) -replace 'Start time: ', ''
}
$end = ''
Get-Content -Path "ScriptLog.txt" | Select-String "End time"
| ForEach-Object {
$end = ($_.Line ) -replace 'End time: ', ''
}
Write-Host $start
Write-Host $end
$startTime = [DateTime]::ParseExact($start, 'yyyyMMddHHmmss', (Get-Culture))
$endTime = [DateTime]::ParseExact($end, 'yyyyMMddHHmmss', (Get-Culture))
$executionTime = $endTime - $startTime
Write-Host "Script execution time: $executionTime"
After executing the script, you’ll find a log file named ScriptLog.txt
in your current directory, containing the script’s output along with the start and stop timestamps.
Then we will retrieve the start and stop timestamps from the log file and take the difference as the execution time.
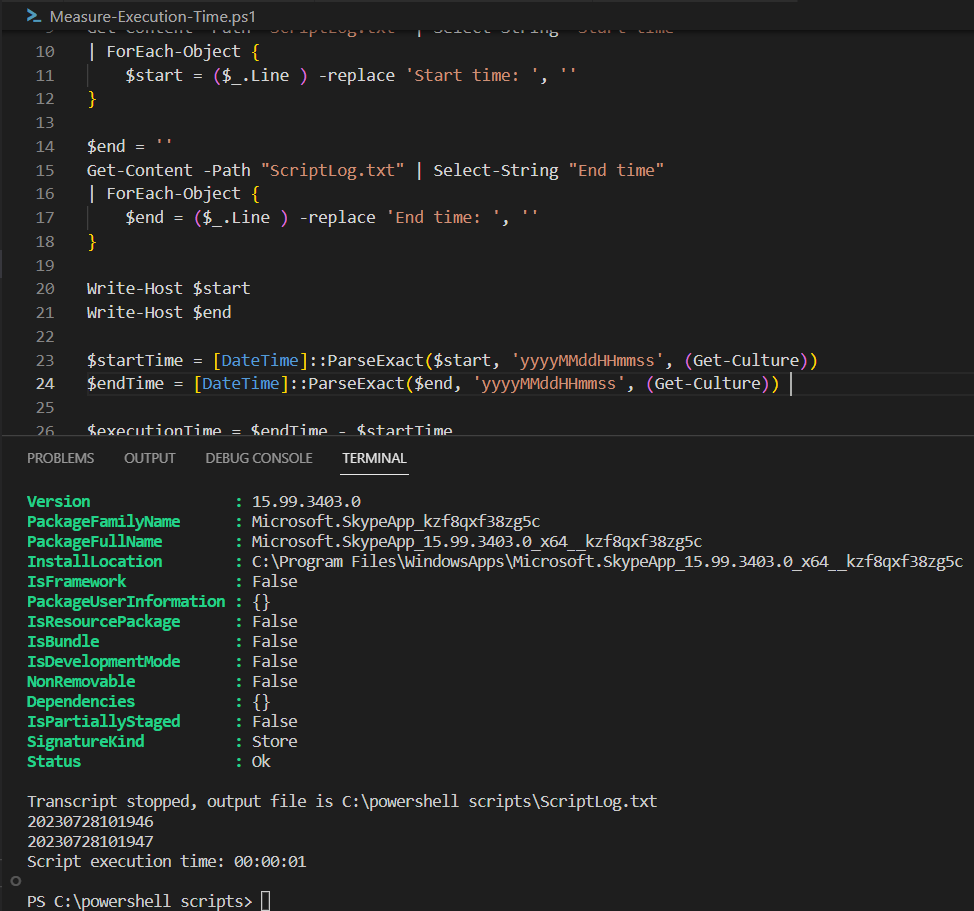
Conclusion
To measure execution time of PowerShell script we can use Measure-Command
and Get-Date
cmdlets.
Since PowerShell is deeply integrated with .NET Framework, we can also use Stopwatch
class from System.Diagnostics.Stopwatch
namespace.
Last, we can also use Start-Transcript
and Stop-Transcript
cmdlets that will record PowerShell session to a log file. Then, we retrieve the start and stop timestamps from the log and take the difference as the execution time.