Copy Files from One Folder to Another using PowerShell
Problem
In this blog post, we will show you many ways to copy files from one folder to another using PowerShell.
As the context, we will copy all powershell script files from C:\Scripts
to C:\powershell scripts
.
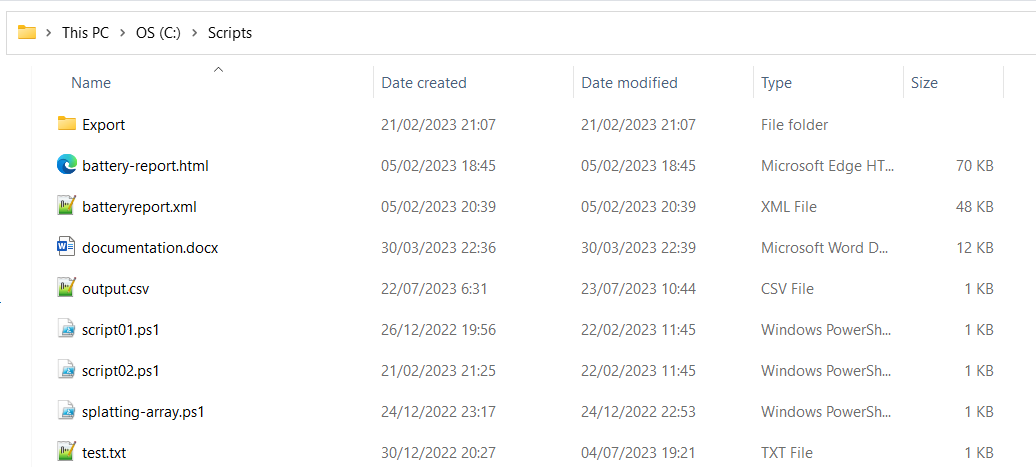
Using Copy-Item Cmdlet
You can use Copy-Item
cmdlet to copy the files from the source folder to the destination folder by specifying -Path
and -Destination
parameters. Each of them represents source and destination folder respectively.
The syntax is as follows:
Copy-Item -Path "Source\file.txt" -Destination "Destination\"
- Replace
Source\file.txt
with the path to the file or files we want to copy. We can use wildcards like*
to copy multiples files, such asSource\*.txt
to copy all.txt
files. - Replace
Destination\
with the path to the destination folder where you want to copy the files. Be sure to include the trailing backslash.
For example, we want to copy all PowerShell script files (.ps1) from C:\Scripts
to C:\powershell scripts
as mentioned earlier, then it will be as follows:
Copy-Item -Path "C:\Scripts\*.ps1" -Destination "C:\powershell scripts\"
If we only want to copy one file, then it will be as follows:
Copy-Item -Path "C:\Scripts\script01.ps1" -Destination "C:\powershell scripts\"
Using Robocopy
Robocopy
is a command-line file transfer utility available in Windows. You can use it from PowerShell to copy files and directories with various options.
Below is the example if we want to copy one file from source to destination folder.
robocopy "C:\Scripts" "C:\powershell scripts\" "script01.ps1"
Below is the example if we want to copy multiple files from source to destination folder.
robocopy "C:\Scripts" "C:\powershell scripts\" "*.ps1"
The result will look as follows where it shows the statistics after successfully copying the files:
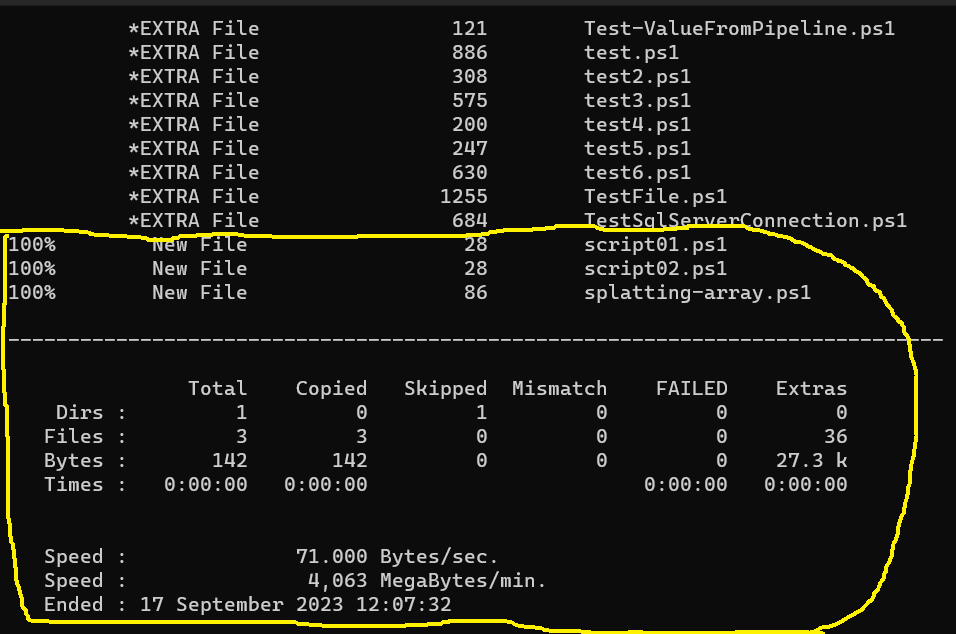
Using Xcopy
Xcopy
is another built-in Windows command for copying files. It’s a simpler option compared to robocopy.
Below is the example if we want to copy one file from source to destination folder. /y
parameter is used to confirm that the file in destination folder will be overwritten.
xcopy "C:\Scripts\script01.ps1" "C:\powershell scripts\" /y
Below is the example if we want to copy multiple files from source to destination folder.
xcopy "C:\Scripts\*.ps1" "C:\powershell scripts\" /y
The result will look as follows:

Conclusion
To copy files from one folder to another in PowerShell, we can use Copy-Item
cmdlet.
There are also other tools available in Windows to achieve the same thing, such as: Robocopy
and Xcopy
. They have various options or parameters that make them powerful