How to Convert String to Datetime in PowerShell
Introduction
Converting string to datetime is a common problem when coding or scripting. In this blog post, we will show you different ways to convert string to datetime in PowerShell.
Solution
Using ParseExact
This method is borrowed from DateTime
class in .NET Framework. This class provides many methods dealing with parsing datetime using specific format. The following example will convert string 20231502-1745 to its datetime form which is 15th February 2023, 17:45.
$string = '20231502-1745'
[DateTime]::ParseExact($string, 'yyyyddMM-HHmm', (Get-Culture))
Using TryParseExact
This method is similar to ParseExact
but is safer to use because we can try whether the string is really a valid datetime or not. If not valid, then we can resort to other logic.
However, if we use ParseExact
and the string is not a valid datetime, then it will throw an error immediately.
$date = Get-Date
$string = '20231502-1745'
if ([DateTime]::TryParseExact($string, 'yyyyddMM-HHmm', $null, 'None', [Ref]$date)) {
$date
}
Using Casting Operator
We can cast the string directly to datetime as follows:
$string = "11/10/2023"
[DateTime]$string
However, there is a problem with using casting operator. By default, it will use culture in our computer. So, in UK the above string will represent 11th October 2023, but in US it will represent 10th November 2023.
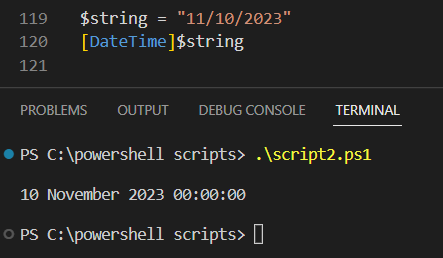
Conclusion
In conclusion, you can use method from .NET Framework such as ParseExact
and TryParseExact
to convert string to datetime. Using TryParseExact
is a safer way to do the conversion.
Using casting operator is also another way to convert, but you should avoid it because it doesn’t take into account cultural difference.