How to Convert String to Integer in PowerShell
Introduction
Converting string to integer can be a tricky process. Fortunately, PowerShell provides an easy way to convert string into integer and other data types. In this blog post, we’ll take a look at how you can use some commands to convert string into integer.
Solution
Using Casting Operator
This method is the easiest and most straightforward way to convert string to integer. This method works just like casting in other programming languages such as C#, Java, etc. Here’s an example of how this would work:
$string = "123"
$num = [int]$string
Write-Host $num
Using Convert class from .NET Framework
The second way that we will discuss is by utilizing the .NET Framework classes provided by Microsoft. These classes provide powerful methods for performing various types of data conversion tasks including converting string into integer.
The most commonly used class is System.Convert
which provides several static methods that are specifically designed for converting string into different data types such as integer or doubles.
To get list of static methods that can be used for conversion, we can use following command:
[Convert] | Get-Member -Static
Later, to convert string to integer, we can use following script:
$string = "123"
$num = [Convert]::ToInt32($string)
Write-Host $num
Integer has various range. Int16 ranges from -32,768
to 32,767
. Int32 ranges from -2,147,483,648
to 2,147,483,647
, while Int64 or long ranges from -9,223,372,036,854,775,808
to 9,223,372,036,854,775,807
.
If we try to convert a string representing a number which is outside the range, then we will get error Value was either too large or too small for an Int32
like below example:
$string = "9223372036854775807"
$num = [Convert]::ToInt32($string)
Write-Host $num
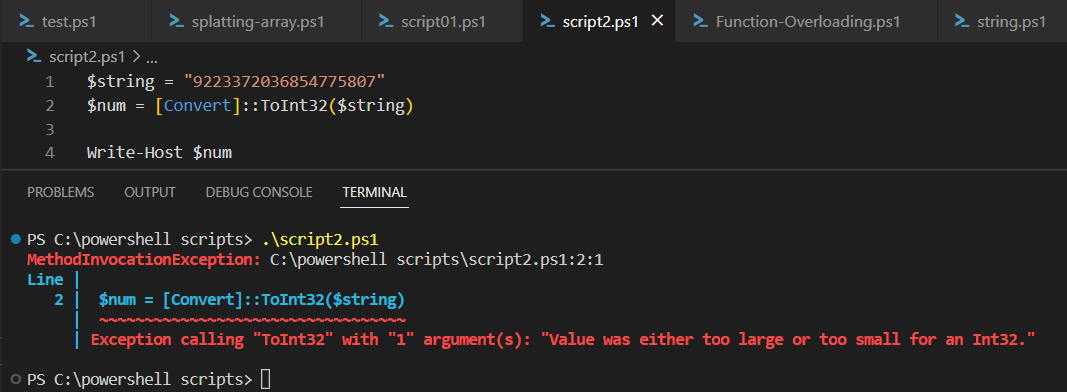
Using Parse Method from .NET Framework Integer Classes
Another .NET Framework class that can be used is Int32
struct. It provides method like Parse
to convert string to integer.
$string = "123"
$num = [Int32]::Parse($string)
Write-Host $num
Using TryParse Method from .NET Framework Integer Classes
Besides Parse
method, Int32
struct also has a safe method to convert string to integer which is TryParse
. This method will check whether the string can be converted to integer or not while at the same time it will out the conversion result. To do this, we must use ref
parameter modifer which is the counterpart of out keyword in C#.
$string = "123"
$num = $null
$success = [Int32]::TryParse($string, [ref]$num)
if ($success) {
Write-Host $num
}
Conclusion
In conclusion, there are several ways to easily convert string into integer in PowerShell. While all methods produce the same result, the last method which is using TryParse
supposed to be the safest way to convert string to integer. It’s because sometimes we do not know whether the string supplied representing a valid integer or not.