How to Handle Error in PowerShell
Introduction
There are 2 type of errors in PowerShell:
Non-terminating Errors
Non-terminating error allows the execution of the script or command to continue when the error occurs. It can act as a warning to user.
How to Raise Non-terminating Errors
To raise non-terminating error, we can use Write-Error
cmdlet in the case where expected error will happen. When the error is raised, PowerShell will highlight the message with certain color (typically red) to differentiate it with console output, but it will not halt the script or command execution.
This can be seen in the following example where we invoke a function that takes input from pipeline. In the middle of execution, there is an input that will raise an error but the execution will still continue until the last input.
function Test-Even {
[CmdletBinding()]
param (
[Parameter(Mandatory, ValueFromPipeline)]
[int]$Value
)
process {
if ($Value % 2 -eq 1) {
Write-Error ('The value {0} is not even number' -f $Value)
}
else {
'The value {0} is an even number' -f $Value
}
}
}
2, 5, 16 | Test-Even
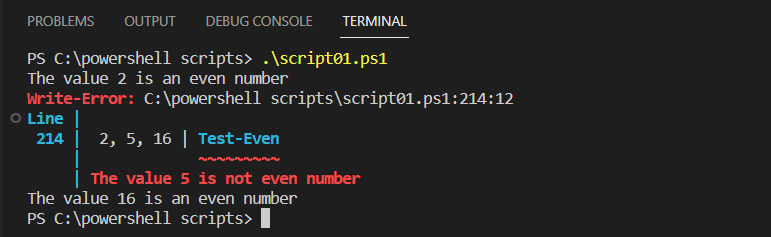
When to Use Non-terminating Errors
Non-terminating errors should be used in situations where the error is not severe enough to warrant stopping the execution of a command or script.
For example, you can apply it for input validation. If your script receives a stream of items that can have different type, you can use non-terminating errors to ensure that the individual input is numeric and within a certain range.
Therefore, besides processing the data you will also get the feedback from the script.
How to Handle Non-terminating Errors
To handle non-terminating errors, you can use the ErrorAction
parameter to control how PowerShell responds to them. By default, the value of this parameter is Continue
.
You can set the ErrorAction
parameter to SilentlyContinue
to suppress the error messages and continue execution. You can even set it to Stop
to make it behave like terminating erros where the execution will stop immediately after the error occurs.
In short, ErrorAction
parameter has following values:
- Break
- Continue
- Ignore
- Inquire
- SilentlyContinue
- Stop
- Suspend
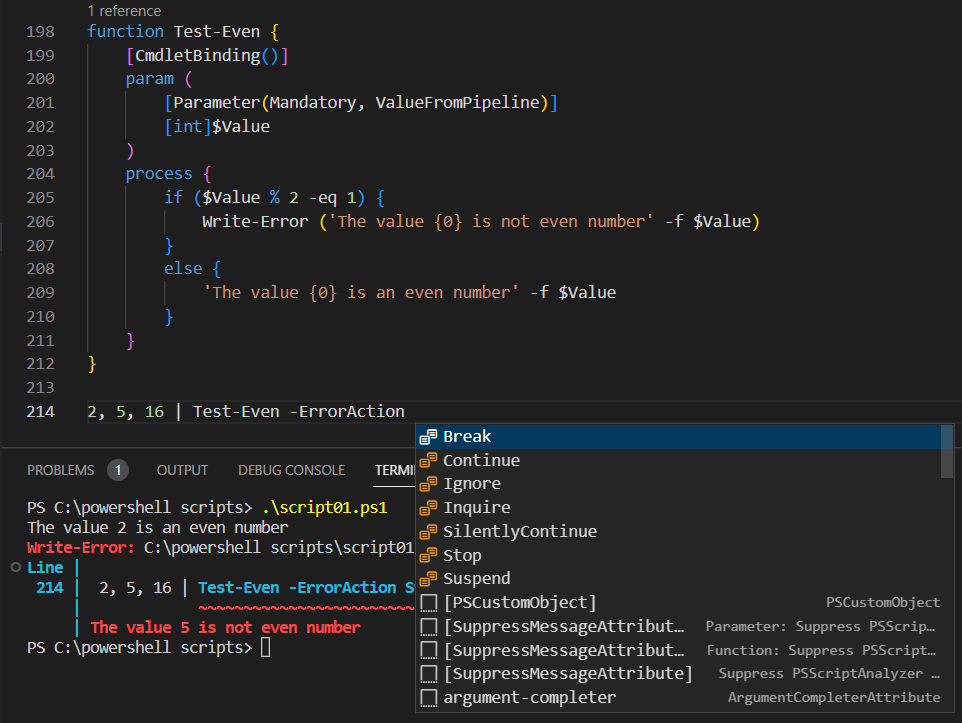
Terminating Errors
Terminating error stops the execution of the script or command immediately after the error occurs. The error is sometimes also called exception error.
How to Raise Terminating Errors
Terminating error can be raised automatically or manually. To manually raise the error, we can use throw
keyword.
Meanwhile terminating erros is raised automatically when there is a logic that breaks the execution. For example, dividing a number by zero will raise an error and halt the execution of the script.
When the error occurs, PowerShell will display an error message in red text and halt the execution of the command or script. The error message will include information about the error, such as the error type, error message, and the line of code where the error occurred.
It’s important to have proper handle for this kind of error.
When to Use Terminating Errors
Terminating errors should be used when the error is severe enough to stop the execution of a command or script. This is typically the case when an error prevents the command or script from completing its intended task or causes unexpected behavior that could lead to data loss or other problems.
Here are some examples of situations where terminating errors may be appropriate:
Critical system errors: If your PowerShell script is performing critical system tasks, such as modifying system files or registry keys, a terminating error should be used if the task cannot be completed successfully. This will prevent the script from making irreversible changes that could cause system instability or data loss.
Data validation errors: If your PowerShell script is processing user input or data from external sources, a terminating error should be used if the data is invalid or does not meet the required format. This will prevent the script from performing actions on invalid data that could lead to unexpected behavior or data corruption.
Security-related errors: If your PowerShell script encounters security-related errors, such as access denied or authentication failures, a terminating error should be used to prevent unauthorized access or data leaks.
How to Handle Terminating Errors
To handle the error, we can use the try...catch
statement to catch the error and perform a specific action in response. For example, you can use the try...catch
statement to display a custom error message or log the error to a file.
The basic syntax of try...catch
is as follows:
try {
# Code that may throw an error
}
catch {
# Code to handle the error
}
finally {
# Code to execute regardless of whether an error occurred or not
}
We can generically catch the errors using catch
clause only:
function Divide-Numbers {
param(
[Parameter(Mandatory = $true)]
[int]$Dividend,
[Parameter(Mandatory = $true)]
[int]$Divisor
)
try {
$Dividend / $Divisor
}
catch {
Write-Host "Error: $_"
}
}
Divide-Numbers -Dividend 10 -Divisor 0
We can also catch specific exception or error generically by specifying the exception name:
function Divide-Numbers {
param(
[Parameter(Mandatory = $true)]
[int]$Dividend,
[Parameter(Mandatory = $true)]
[int]$Divisor
)
try {
$Dividend / $Divisor
}
catch [System.DivideByZeroException] {
Write-Host "Error: $_"
}
}
Divide-Numbers -Dividend 10 -Divisor 0
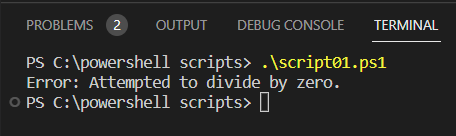
We could also have multiple catch
clauses to respond different type of exceptions.
Conclusion
There are two kinds of error: non-terminating error and terminating error. Non-terminating error can be raised using Write-Error
cmdlet while terminating error can be raised by using throw
keyword if it’s manually raised.
In response to the error, we also need proper error handling. Without proper error handling, PowerShell scripts may continue running after encountering errors as if everything is normal. It can also provide informative error messages to users, making it easier for them to debug what went wrong and take appropriate action.