Rename Excel Workbook using PowerShell
Problem
In this blog post, we will show you how to rename Excel workbook using PowerShell. As we know, workbook is the other name of excel file, so we can simply call it excel file.
As the context, the name of our original workbook is Test.xlsx
as follows:
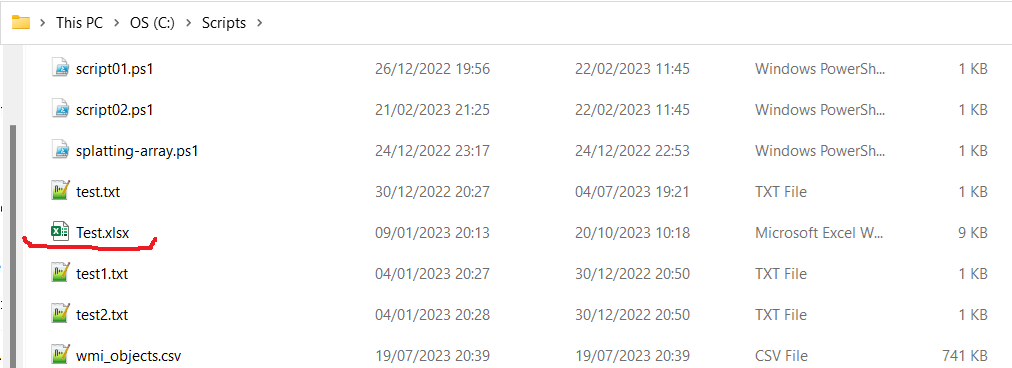
And we will rename it to be Book.xlsx
.
Using Excel Libraries
There are many excel libraries that can be used to rename excel workbook, from Excel Com Object
which stems from .NET Framework to external PowerShell modules such as ImportExcel
and PSExcel
. All these libraries/modules allow us to manipulate Excel object.
The approach we use here is saving excel object to a new file and then removing the old file.
Using Excel Com Object
After creating the object, we will open the Excel file to get the workbook we want to manipulate. Then, we save the existing workbook as a new file using SaveAs
method before removing the old file.
try {
# Define old and new excel files
$oldFile = "C:\Scripts\Test.xlsx"
$newFile = "C:\Scripts\Book.xlsx"
# Create excel object
$excel = New-Object -ComObject Excel.Application
$workbook = $excel.Workbooks.Open($oldFile)
# Save excel file to a new file
$workbook.SaveAs($newFile)
# Remove original file
Remove-Item -Path $oldFile
}
finally {
# Close excel file
$workbook.Close()
$excel.Quit()
[System.Runtime.Interopservices.Marshal]::ReleaseComObject($excel)
}
We enclose the code above with try-finally
block because we want to make sure it will release the resource after modifying Excel that is performed at finally
block.
Using ImportExcel Module
ImportExcel
is an external module that is built based on EPPlus which is a very well-known library to work with Excel spreadsheets in .NET.
Before using ImportExcel
module, we have to install it.
Install-Module -Name ImportExcel
And then following previous solution pattern, we have to create Excel object first before saving the existing excel object to a new file using SaveAs
method. Then, we remove the old file.
try {
# Import the module
Import-Module ImportExcel
# Define old and new excel files
$oldFile = "C:\Scripts\Test.xlsx"
$newFile = "C:\Scripts\Book.xlsx"
# Create excel object
$excel = Open-ExcelPackage -Path $oldFile
# Save excel file to a new file
$excel.SaveAs($newFile)
# Remove original file
Remove-Item -Path $oldFile
}
finally {
# Close excel file
Close-ExcelPackage $excel
}
Similar to previous solution, we enclose the script with try-finally
block to avoid memory leak.
Using PSExcel Module
PSExcel
is another module based on EPPlus library. You can find all the examples in github repository.
Before using this module, we have to install it.
Install-Module -Name PSExcel
In order to use the object, we must import the module first. Then, we create Excel object by specifying the path of our Excel file. We will save this object as a new file using Save-Excel
cmdlet from this module and then remove the old file.
try {
# Import the module
Import-Module PSExcel
# Define old and new excel files
$oldFile = "C:\Scripts\Test.xlsx"
$newFile = "C:\Scripts\Book.xlsx"
# Create excel object
$excel = New-Excel -Path $oldFile
# Save excel file to a new file
Save-Excel -Excel $excel -Path $newFile
# Remove original file
Remove-Item -Path $oldFile
}
finally {
$excel | Close-Excel
}
We also enclose the script with try-finally
block to avoid memory leak.
Using PowerShell Cmdlet
Since workbook is essentially an excel file, we can rename the file using PowerShell cmdlets.
Using Rename-Item Cmdlet
Rename-Item
is built-in PowerShell cmdlet to rename a file.
# Define old excel file
$oldFile = "C:\Scripts\Test.xlsx"
# Define new excel file
$newFile = "C:\Scripts\Book.xlsx"
# Rename the file
Rename-Item -Path $oldFile -NewName $newFile
Using Move-Item Cmdlet
Move-Item
cmdlet can also be used to rename the file by moving the file to the same location but different name, hence renaming the file.
# Define old excel file
$oldFile = "C:\Scripts\Test.xlsx"
# Define new excel file
$newFile = "C:\Scripts\Book.xlsx"
# Move the file to the new name/location, hence rename the file
Move-Item -Path $oldFile -Destination $newFile
Using System.IO.File class
Since PowerShell allows us to use .NET Framework class, we can use Move
method from System.IO.File
class to rename the file using similar approach in previous solution.
# Define old excel file
$oldFile = "C:\Scripts\Test.xlsx"
# Define new excel file
$newFile = "C:\Scripts\Book.xlsx"
# Rename the file using .NET Framework System.IO.File class
[System.IO.File]::Move($oldFile, $newFile)
Conclusion
To rename excel workbook using PowerShell, we can use excel module/libraries to manipulate excel object.
We can use Excel Com Object
which is based on .NET Framework. We can also use PowerShell external modules like PSExcel
and ImportExcel
. ImportExcel
is an excellent module which is based on EPPlus
library, a well-known C# libary for woking with Excel from .NET.
PSExcel
is the alternative of ImportExcel
module. PSExcel
is also based on EPPlus
but this module is no longer maintained as stated in its GitHub repository. If it doesn’t provide functionality that you need, you should contribute to the source code by yourself.
We can also use PowerShell cmdlets to rename the workbook because it is essentially the same with renaming Excel file. We can use Rename-Item
, Move-Item
cmdlets as well as Move
method from System.IO.File
class.