How to Empty Recycle Bin using PowerShell
Introduction
In this blog post, we will walk through how to empty recycle bin in PowerShell.
Solution
All solutions provided below has the same purpose. However, there is a caveat for methods other than using Clear-RecycleBin
cmdlet where the bin status won’t be reflected in the Explorer (the desktop icon) until you actually open the Recycle Bin and/or refresh the desktop.
Using Clear-RecycleBin Cmdlet
PowerShell has native command/cmdlet which is Clear-RecycleBin
to empty recycle bin.
You can use below command to empty recycle bin:
Clear-RecycleBin
After performing above command, it will ask you to confirm whether you want to delete all the contents of Recycle Bin as in following image:
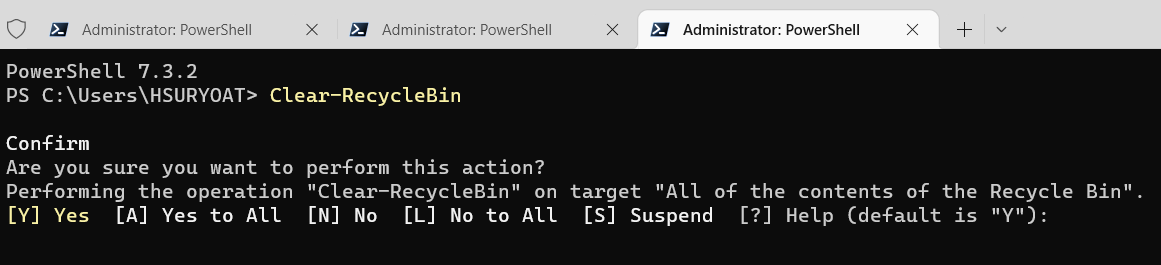
To avoid being asked for confirmation, you can use Force
switch parameter.
Clear-RecycleBin -Force
To understand more about this cmdlet like the available parameters to use, you can check the help as in following image:
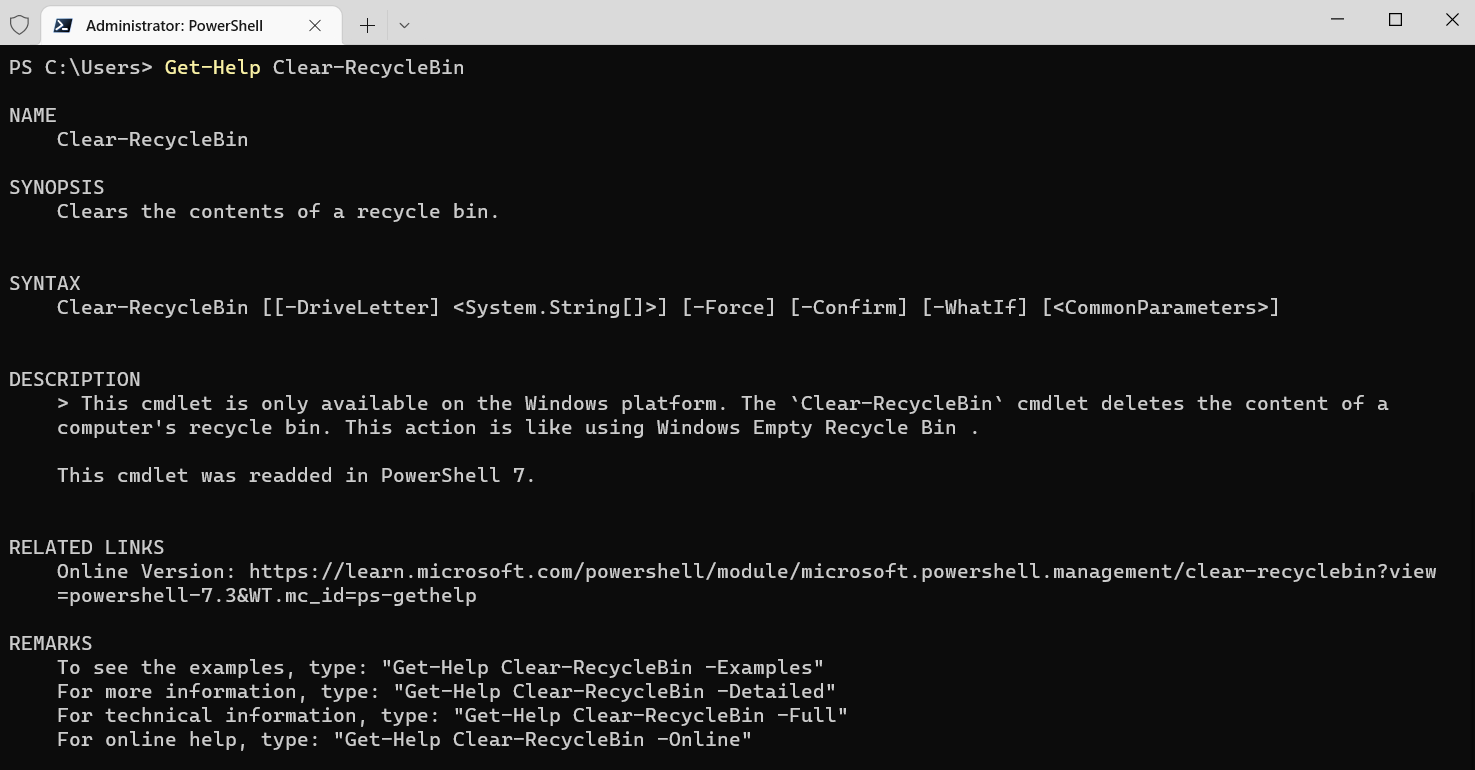
Empty recycle bin for specific drive using Clear-RecycleBin cmdlet
When using Clear-RecycleBin
cmdlet, you can also specify the drive you want to empty. Suppose you want to empty drive C
only, then you have to use DriveLetter
parameter as in following example:
Clear-RecycleBin -DriveLetter 'C'
Using Remove-Item cmdlet combined with System Drive environment variable
Theoretically, all deleted items are stored in system drive where it has hidden location/directory with the name $Recycle.bin
. So, besides using native cmdlet Clear-RecycleBin
, we can also try to seek this hidden directory then delete the contents as in following script:
$driveName = (Get-ChildItem -Path Env:\SystemDrive).Value
Remove-Item -Path $driveName\`$recycle.bin -Recurse -Force
The above script will get the system drive from environment variable first before applying it to remove-item
cmdlet to delete the contents.
The script also has counterpart in Windows Command Prompt which is rd /s /q %systemdrive%\$Recycle.bin
that will serve the same purpose.
Using Shell.Application from .NET Framework
In PowerShell, we can also create object from .NET Framework classes. So we can also use C# code to empty recycle bin.
$Shell = New-Object -ComObject Shell.Application
$RecBin = $Shell.Namespace(0xA)
$RecBin.Items() | ForEach-Object { Remove-Item $_.Path -Recurse -Confirm:$false }
Conclusion
In conclusion, to empty recycle bin in PowerShell, we can use PowerShell Clear-RecycleBin
native cmdlet.
Besides that, we can also use Remove-Item
cmdlet that must be combined with system drive environment variable to find the hidden directory of recycle bin where it stores all deleted items.
Last, as usual we can also use .NET Framework classes to achieve our task in PowerShell. In this case, we utilize Shell.Application
ComObject from C#.