How to Add Property to PSCustomObject in PowerShell
Problem
In PowerShell, PSCustomObject allows us to create an object on the fly without having to create a class and its members such as Properties, Methods, etc.
This capability makes PSCustomObject an ideal solution for some purposes like combining data into one object and dynamically change the property and behavior of an object.
In this blog post, we will show you several ways to add property to PSCustomObject in PowerShell.
Using Add-Member cmdlet and NoteProperty
You can use Add-Member
cmdlet to add property to PSCustomObject.
In the following example, we create a PSCustomObject called $fullName
that initially consists only of First and Last name. Then, we use Add-Member
cmdlet to add new property called MiddleName
with value Quincy
that will represent person middle name.
NoteProperty
denotes a generic property that will be attached to an object. Finally, we can access the newly added MiddleName
property using $fullName.MiddleName
.
# Create a PSCustomObject
$fullName = [PSCustomObject]@{
FirstName = "John"
LastName = "Adam"
}
# Add a new property to the object
$fullName | Add-Member -MemberType NoteProperty -Name "MiddleName" -Value "Quincy"
# Access the new property
$fullName.MiddleName
The result will look as follows:
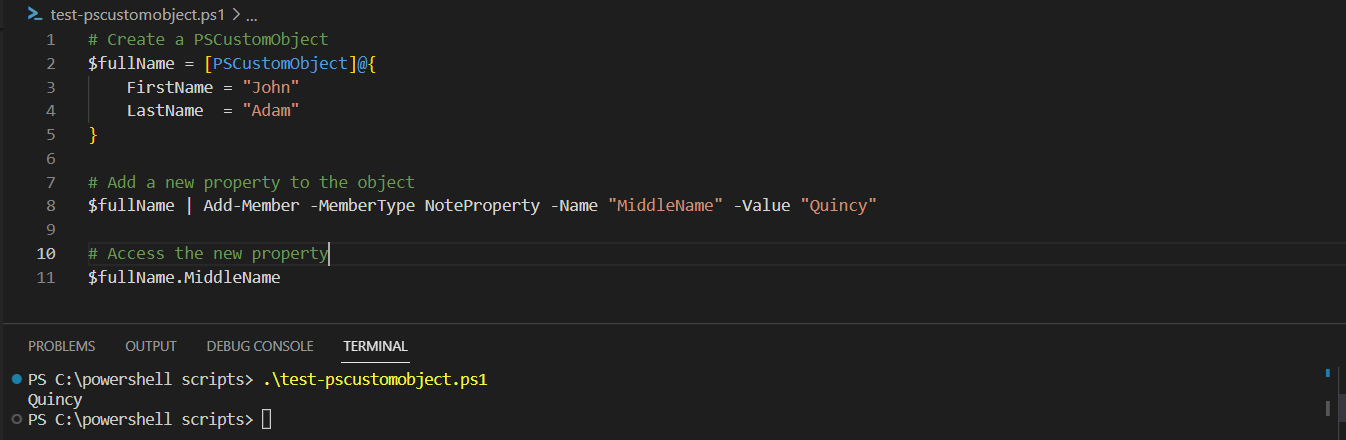
Using Calculated Properties
In this approach, we use the Select-Object
cmdlet to create a new object based on the existing $fullName
object.
We specify *
to include all existing properties, and then we define a new calculated property using the @{}
syntax. The new calculated property is named MiddleName
, and its expression is set to Quincy
.
By assigning the result of Select-Object
back to $fullName
, we update the object with the newly added property. Finally, we can access the MiddleName
property using $fullName.MiddleName
.
# Create a PSCustomObject
$fullName = [PSCustomObject]@{
FirstName = "John"
LastName = "Adam"
}
# Add a new property to the object using Select-Object
$fullName = $fullName | Select-Object *, @{Name = "MiddleName"; Expression = { "Quincy" } }
# Access the new property
$fullName.MiddleName
The result will look as follows:
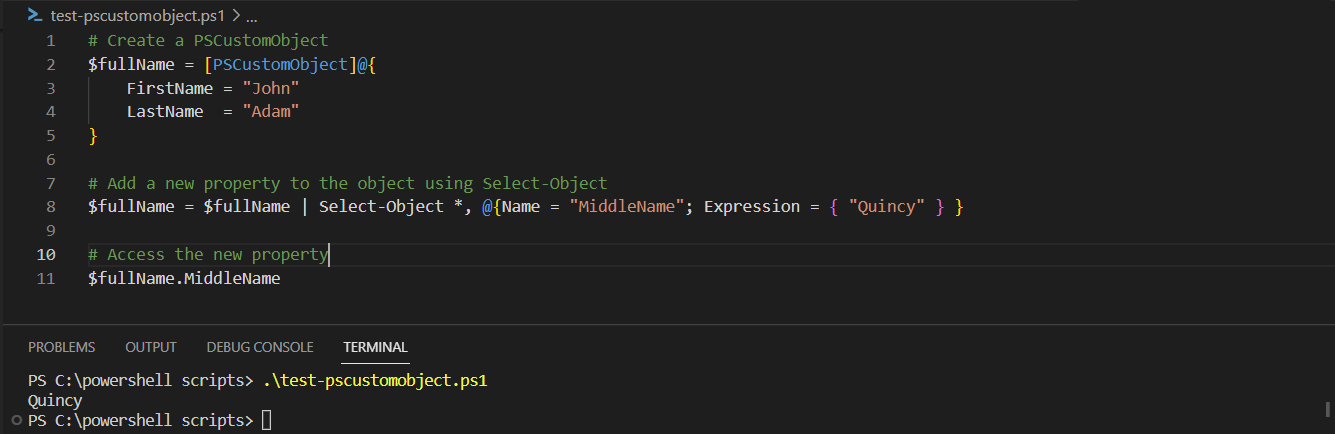
Using Add method
In this approach, we access the psobject
property of the $fullName
object, which provides access to the underlying object properties. We then use the Properties.Add()
method to add a new property to the object. The PSNoteProperty
class is used to define the new property with a name MiddleName
and value Quincy
.
Once the property is added, we can access it using $fullName.MiddleName
.
# Create a PSCustomObject
$fullName = [PSCustomObject]@{
FirstName = "John"
LastName = "Adam"
}
# Add a new property to the object using the Add() method
$fullName.psobject.Properties.Add([PSNoteProperty]::new("MiddleName", "Quincy"))
# Access the new property
$fullName.MiddleName
The result will look as follows:
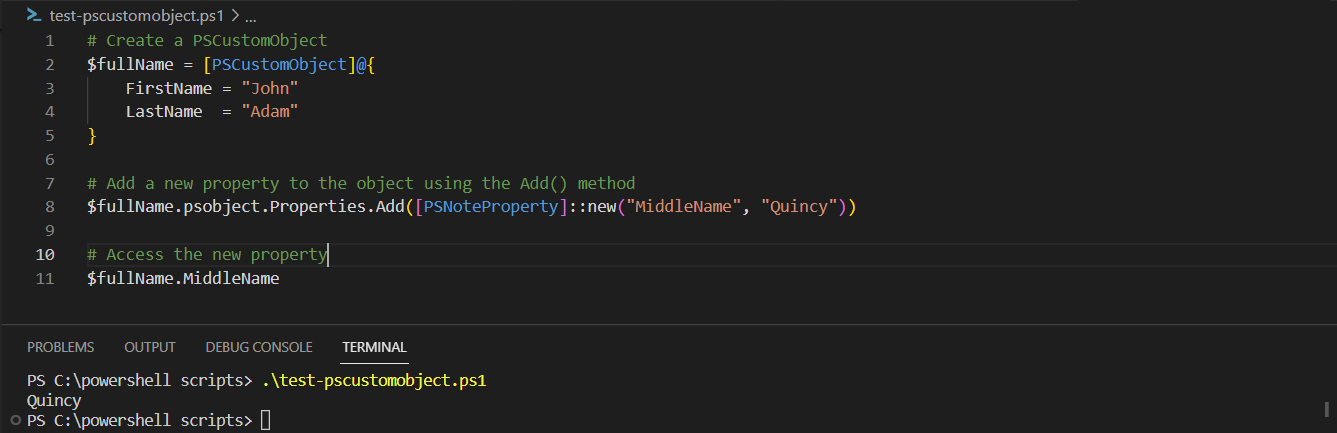
Conclusion
To add property to PSCustomObject, we can use the combination of Add-Member cmdlet
and NoteProperty
. We can also use Calculated Properties. Last, we can use Add
method from psobject
which is an intrinsic member of PSCustomObject.