How to Pass Function as a Parameter
Introduction
In PowerShell, you can pass function as a parameter to another function. This is similar to capability in other programming languages where you can create Higher-order function through lambda or anonymous function.
Problem
When working with PowerShell, sometimes you want to pass function as parameter to another function, then you want to invoke the passed function.
Solution
To pass the function as parameter, you can pass it the same way like passing a value. In this case, the function will be the value. Then, you can invoke the passed function using &
(the call operator).
Let’s take a look at some examples.
Passing a Function without Parameters
You can pass the function by position, name or Script Blocks
. In other programming languages, Script Blocks
is equivalent to anonymous function or lambda.
function MyFunction {
Param($param)
Write-Host "I'm calling: $(& $param)"
}
Function Test {
"Function Test"
}
MyFunction Test
MyFunction -param Test
MyFunction -param { Test }
Below is the output if we execute above script.
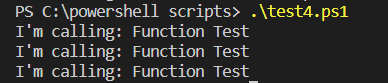
Passing a Function with Parameters
To pass function with its parameters, you can use args
automatic variable to get the arguments of passed function.
function MyFunction {
Param($param)
Write-Host "I'm calling: $(& $param @args)"
}
Function Test {
"Function Test with parameters: $args"
}
MyFunction Test a b
MyFunction -param Test a b
MyFunction -param { Test @args } a b
The last line of code above which is MyFunction -param { Test @args } a b
using splatting to pass the arguments stored in the args
automatic variable.
Below is the output if we execute above script.

Conclusion
In order to pass function as parameter, the mechanism is the same with passing a value. You can pass it by the name, position or Script Blocks
. If you want to invoke the passed function, you can use call operator (&
), and also if you want to pass the function with its arguments you can use args
automatic variable.