Open Edge Browser with Url from C#
Introduction
Having the ability to open Edge browser from C# is extremely useful. It can save you time and energy by allowing you to quickly open Edge browser with specific URL without having to open and type the URL manually.
In this article, we will show you many ways to open Edge browser with URL from C#.
Using Process.Start() method
To use this method, we have to include using System.Diagnostics;
directive in our code. Then, we use Process.Start()
method to launch the browser and open specific url.
But, there is a caveat when using this method that it behaves differently between .NET Framework and .NET Core.
In .NET Framework, by default Process.Start()
will set UseShellExecute
as true behind the scene while in .NET Core, UseShellExecute
will be set as false. So, you must be aware of the platform chosen.
.NET Framework
The following code will work in .NET Framework but not in .NET Core.
using System.Diagnostics;
namespace ConsoleApp1
{
internal class Program
{
static void Main(string[] args)
{
Process.Start("microsoft-edge:http://www.byteinthesky.com");
}
}
}
The alternative code in .NET Framework is as follows:
string browser = "msedge.exe";
string url = "http://www.byteinthesky.com";
Process.Start(browser, url);
.NET Core
If you use .NET Core, the workaround is to explicitly specify browser executable file:
string browser = @"C:\Program Files (x86)\Microsoft\Edge\Application\msedge.exe";
string url = "http://www.byteinthesky.com";
Process.Start(browser, url)
But, in case we don’t want to know browser executable file location, we must use ProcessStartInfo
and set UseShellExecute
to be true:
ProcessStartInfo processStartInfo = new ProcessStartInfo
{
UseShellExecute = true,
FileName = "http://www.byteinthesky.com",
};
Process.Start(processStartInfo);
The code above will open the website using default browser.
To allow us open the url in different browser, we can set the browser to FileName
property and the url to Arguments
property as follows:
Process.Start(new ProcessStartInfo()
{
UseShellExecute = true,
FileName = "chrome",
Arguments = "http://www.byteinthesky.com"
});
The chrome
could be replaced with any browsers like msedge
, firefox
, iexplore
that can be resolved by operating system.
Using CMD
We can utilize command prompt (CMD) to open Microsoft Edge browser. The effect of this code will be the same as if we execute start msedge "http://www.byteinthesky.com"
from command prompt.
using System.Diagnostics;
namespace ConsoleApp1
{
internal class Program
{
static void Main(string[] args)
{
string url = "http://www.byteinthesky.com";
Process.Start("CMD.exe", $"/C start msedge {url}");
}
}
}
Invoke Powershell Script from C#
This approach will launch Edge browser through PowerShell since Microsoft provides libraries to programmatically work with PowerShell. You need to install these packages from Nuget.
Microsoft.PowerShell.SDK
System.Management.Automation
Below C# code will compose PowerShell script that will be equivalent to Start-Process msedge "http://www.byteinthesky.com"
command. The Invoke
method will launch the default program that will open the url on Edge browser.
using System.Collections.ObjectModel;
using System.Management.Automation;
namespace ConsoleApp3
{
internal class Program
{
static void Main(string[] args)
{
PowerShell ps = PowerShell.Create();
ps.AddCommand("Start-Process");
ps.AddArgument("msedge");
ps.AddArgument(@"http://www.byteinthesky.com");
ps.Invoke();
}
}
}
We can replace msedge
with any browsers that can be resolved by operating system like chrome
, firefox
, iexplore
, etc.
As of this article’s date, we use .NET 6 for C#. There are some version of both packages that works well with .NET 6. One of them is 6.2.7
. If you use .NET 7, likely you have to use version 7 onwards. So, you have to be aware regarding packages version and .NET version that you are using.
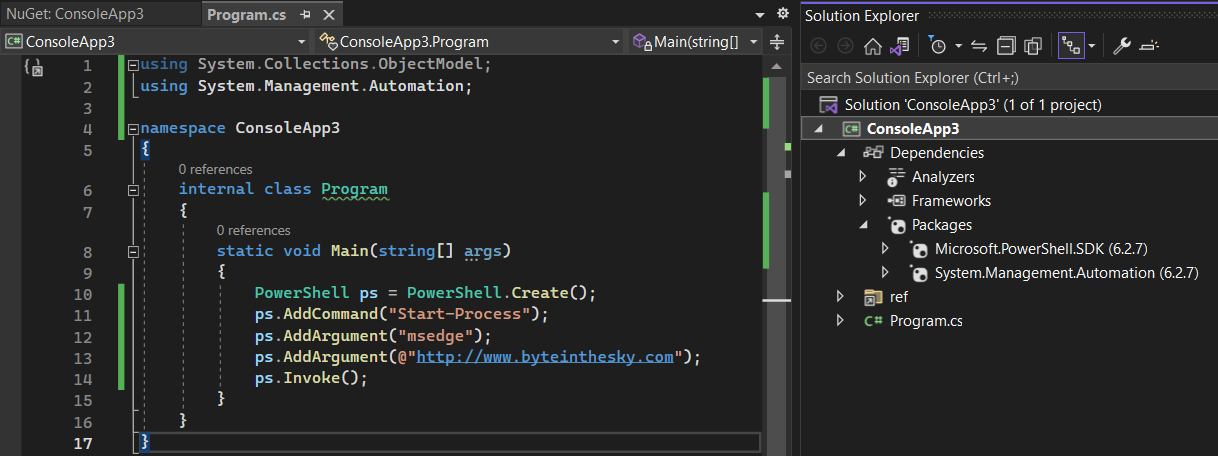
Using CliWrap Package
The CliWrap
package makes working with CLIs in C# easy and powerful. It works with all CLIs like powershell
, cmd
, git
, npm
, docker
, etc.
This example focuses on PowerShell and compose a PowerShell script which will execute Start-Process msedge "http://www.byteinthesky.com"
command behind the scene.
We need to add a reference to the CliWrap
package in our project and then add the code to call our PowerShell script.
using CliWrap;
using CliWrap.Buffered;
namespace ConsoleApp4
{
internal class Program
{
static async Task Main(string[] args)
{
var dbDailyTasks = await Cli.Wrap("powershell")
.WithArguments(new string[]
{
"Start-Process",
"msedge",
@"http://www.byteinthesky.com"
})
.ExecuteBufferedAsync();
Console.WriteLine(dbDailyTasks.StandardOutput);
Console.WriteLine(dbDailyTasks.StandardError);
Console.ReadLine();
}
}
}
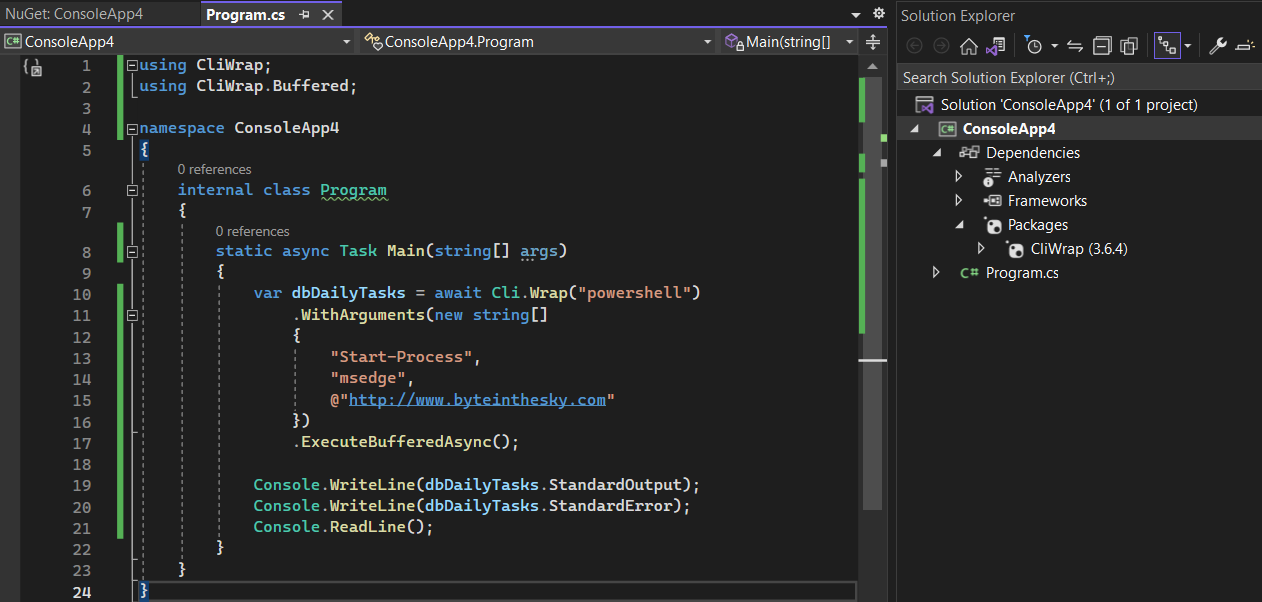
Conclusion
There are many ways to open Edge browser with url using C#. C# has built-in library to spawn a process to launch the browser in System.Diagnostics
namespace. We could use Process.Start
method and also combine this method with ProcessStartInfo
.
We could also utilize command prompt. Then, we can also use Microsoft.PowerShell.SDK
and System.Management.Automation
allowing us to write a code that will open a web page on Edge browser.
Last, we can also use CliWrap
package that allows us to execute any CLIs command, not limited to PowerShell, through C#.