Open Text File in Notepad Using C#
Introduction
Having the ability to open text file to Notepad from C# is extremely useful. It can save you time and energy by allowing you to quickly edit text files without opening the program manually.
In this article, we will show you many ways to open text file in notepad using C#.
Using Process.Start() method
To use this method, we have to include using System.Diagnostics;
directive in our code.
using System.Diagnostics;
namespace ConsoleApp1
{
internal class Program
{
static void Main(string[] args)
{
string pathToFile = @"C:\Scripts\test.txt";
Process.Start(@"notepad.exe", pathToFile);
}
}
}
This approach assumes notepad.exe
is the default program to open the text files which is typically true. Otherwise, we have to specify the path of the application that will open the file. For example, we could also use Notepad++
to open text file.
string applicationName = @"C:\Program Files\Notepad++\notepad++.exe";
string pathToFile = @"C:\Scripts\test.txt";
Process.Start(applicationName, pathToFile);
Using ProcessStartInfo class
If we don’t care what application will open the text file, we can let the default application to open the file by utilizing UseShellExecute
property of ProcessStartInfo
class.
using System.Diagnostics;
namespace ConsoleApp1
{
internal class Program
{
static void Main(string[] args)
{
string pathToFile = @"C:\Scripts\test.txt";
Process process = new Process();
process.StartInfo = new ProcessStartInfo()
{
UseShellExecute = true,
FileName = pathToFile
};
process.Start();
process.WaitForExit();
}
}
}
If the default application is Notepad++
, then the text file will be opened on Notepad++
.
By setting the UseShellExecute
property to true
, Windows will utilize the default program associated with the file type being opened.
While implementing WaitForExit
will make your application pause until the launched application has been terminated.
Using Windows API ShellExecute function
using System.Runtime.InteropServices;
namespace ConsoleApp1
{
internal class Program
{
// Import the necessary Windows API functions
[DllImport("shell32.dll", CharSet = CharSet.Auto)]
public static extern int ShellExecute(IntPtr hwnd, string lpOperation, string lpFile, string lpParameters, string lpDirectory, int nShowCmd);
static void Main(string[] args)
{
string pathToFile = @"C:\Scripts\test.txt";
try
{
// Use ShellExecute to open the text file in Notepad
ShellExecute(IntPtr.Zero, "open", "notepad.exe", pathToFile, null, 1);
}
catch (Exception ex)
{
Console.WriteLine("An error occurred: " + ex.Message);
}
}
}
}
In this approach, we declare the ShellExecute
function from the shell32.dll
library using the DllImport
attribute. We then use this function to open Notepad
and pass the path of the text file as a parameter.
Note that the ShellExecute
function returns a value indicating the success or failure of the operation. In this example, we’re not capturing or handling the return value, but you can modify the code to handle it as per your requirements.
Make sure to replace C:\Scripts\test.txt
with the actual path and filename of the text file you want to open.
Remember to include the using System.Runtime.InteropServices;
directive at the beginning of your code to access the DllImport
attribute.
Invoke Powershell Script from C#
Microsoft provides libraries to programmatically work with PowerShell. You need to install these packages from Nuget.
Microsoft.PowerShell.SDK
System.Management.Automation
Below C# code will compose PowerShell script that will be equivalent to Start-Process 'notepad.exe' 'C:\Scripts\test.txt'
command. The Invoke
method will launch the default program that will open the text file.
using System.Collections.ObjectModel;
using System.Management.Automation;
namespace ConsoleApp1
{
internal class Program
{
static void Main(string[] args)
{
PowerShell ps = PowerShell.Create();
ps.AddCommand("Start-Process");
ps.AddArgument("notepad.exe");
ps.AddArgument(@"C:\Scripts\test.txt");
Collection<PSObject> results = ps.Invoke();
}
}
}
As of this article’s date, we use .NET 6 for C#. There are some version of both packages that works well with .NET 6. One of them is 6.2.7
. If you use .NET 7, likely you have to use version 7 onwards. So, you have to be aware regarding packages version and .NET version that you are using.
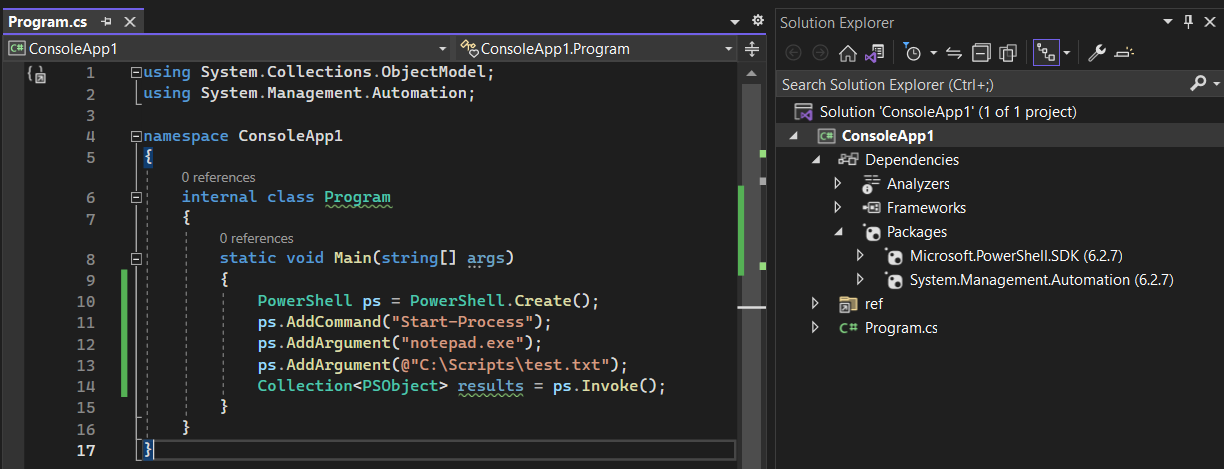
Using CliWrap Package
The CliWrap
package makes working with CLIs in C# easy and powerful. It works with all CLIs like powershell
, git
, npm
, docker
, etc.
This example focuses on PowerShell and calls a PowerShell script which will invoke Start-Process 'notepad.exe' 'C:\Scripts\test.txt'
command inside it.
We need to add a reference to the CliWrap
package in our project and then add the code to call our PowerShell script.
using CliWrap;
using CliWrap.Buffered;
namespace ConsoleApp1
{
internal class Program
{
static async Task Main(string[] args)
{
var dbDailyTasks = await Cli.Wrap("powershell")
.WithArguments(new string[] { "Start-Process", "'notepad.exe'", "'C:\\Scripts\\test.txt'" })
.ExecuteBufferedAsync();
Console.WriteLine(dbDailyTasks.StandardOutput);
Console.WriteLine(dbDailyTasks.StandardError);
Console.ReadLine();
}
}
}
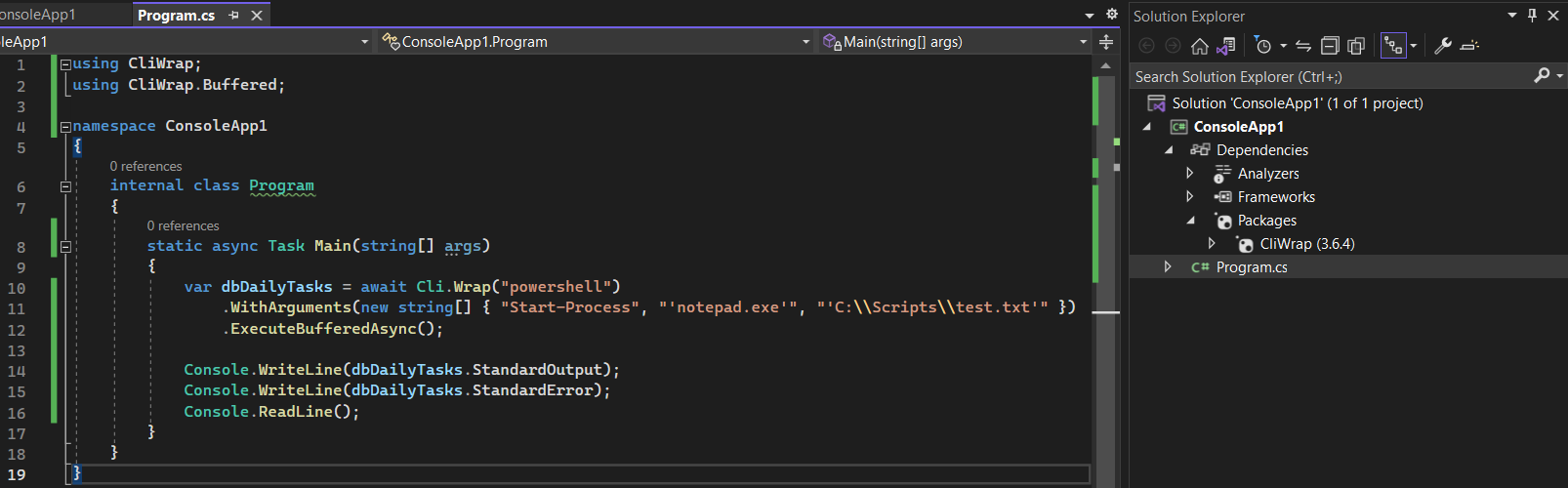
Conclusion
There are many ways to open text file in Notepad using C#. C# has built-in library to spawn a process to open Notepad in System.Diagnostics
namespace. We could use Process.Start
method and also combine this method with ProcessStartInfo
.
We could also utilize Windows API function like ShellExecute. Then, we can use Microsoft.PowerShell.SDK
and System.Management.Automation
allowing us to write a code that will open the text file in Notepad.
Last, we can also use CliWrap
package that allows us to execute any CLIs command, not limited to PowerShell, through C#.