How to Get Edge Version using C#
Introduction
Knowing Edge version is important to ensure its compatibility with other technologies. For example, Selenium Edge WebDriver is tied to Edge version.
If we want to perform web automation on Edge using Selenium, we must be able to detect Edge version so that the automation scripts can download the correct version of the Edge webdriver.
In this article, we will show you how to get Edge version using C#.
Using RegistryKey Class
Information about Edge version can be found in Windows Registry Key located at HKEY_CURRENT_USER\Software\Microsoft\Edge\BLBeacon
.
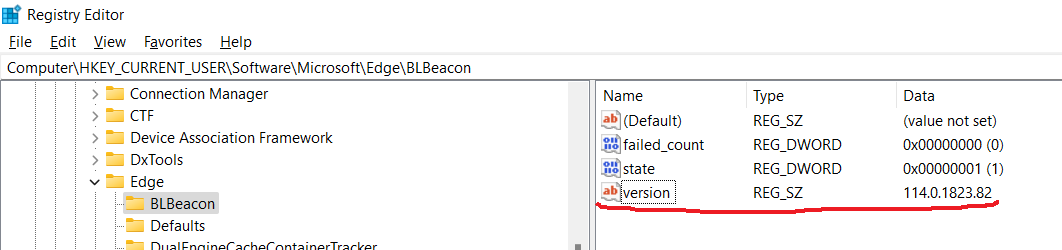
To retrieve Windows Registry, we can use RegistryKey
class from Microsoft.Win32
namespace. Then, we can use OpenSubKey
method to open the registry key.
using Microsoft.Win32;
namespace ConsoleApp5
{
internal class Program
{
static void Main(string[] args)
{
string edgeVersion = GetEdgeVersion();
Console.WriteLine($"Edge version: {edgeVersion}");
}
public static string GetEdgeVersion()
{
string edgeVersion = string.Empty;
const string edgeRegistryKey = @"SOFTWARE\Microsoft\Edge\BLBeacon";
const string edgeRegistryValue = "version";
using (RegistryKey key = Registry.CurrentUser.OpenSubKey(edgeRegistryKey))
{
if (key != null)
{
edgeVersion = key.GetValue(edgeRegistryValue)?.ToString();
}
}
return edgeVersion;
}
}
}

Using FileVersionInfo Class
We could also get Edge version information from executable file located at its installation folder. In this case, the file resides at C:\Program Files (x86)\Microsoft\Edge\Application\msedge.exe
.
using System.Diagnostics;
namespace ConsoleApp7
{
internal class Program
{
static void Main(string[] args)
{
string edgeVersion = GetEdgeVersion();
Console.WriteLine($"Edge version: {edgeVersion}");
}
public static string GetEdgeVersion()
{
string edgeVersion = string.Empty;
string edgeExePath = Path.Combine(Environment.GetFolderPath(Environment.SpecialFolder.ProgramFilesX86), @"Microsoft\Edge\Application\msedge.exe");
if (File.Exists(edgeExePath))
{
FileVersionInfo fileVersionInfo = FileVersionInfo.GetVersionInfo(edgeExePath);
edgeVersion = fileVersionInfo.FileVersion;
}
return edgeVersion;
}
}
}
In this solution, we construct the file path to the Microsoft Edge executable (msedge.exe
) using the Environment.GetFolderPath()
method and the known path pattern. Then, we check if the file exists. If it does, we retrieve the file version information using FileVersionInfo.GetVersionInfo()
.
The GetEdgeVersion()
method returns the version string of Microsoft Edge if the executable file is found and the version information is accessible. Otherwise, it returns an empty string.
Please note that this solution assumes a default installation location for Microsoft Edge. If the installation path or file name changes in future versions, the code may need to be adjusted accordingly.

Using PowerShell Script
Since PowerShell is deeply integrated with .NET Framework, i.e. C#, we can execute PowerShell script from C#.
using CliWrap;
using CliWrap.Buffered;
namespace ConsoleApp7
{
internal class Program
{
static async Task Main(string[] args)
{
string edgeVersion = await GetEdgeVersion();
Console.WriteLine($"Edge version: {edgeVersion}");
}
public static async Task<string> GetEdgeVersion()
{
var dbDailyTasks = await Cli.Wrap("powershell")
.WithArguments(new string[] { @"Get-ItemPropertyValue -Path 'HKCU:\SOFTWARE\Microsoft\Edge\BLBeacon' -Name ""version""" })
.ExecuteBufferedAsync();
return dbDailyTasks.StandardOutput.Trim();
}
}
}
In this case, we use CliWrap
package that enables us to work with any CLIs in C# like powershell
, cmd
, git
, npm
, docker
, etc.
In the above code, we create C# code that will execute PowerShell script Get-ItemPropertyValue -Path 'HKCU:\SOFTWARE\Microsoft\Edge\BLBeacon' -Name "version"
behind the scene.
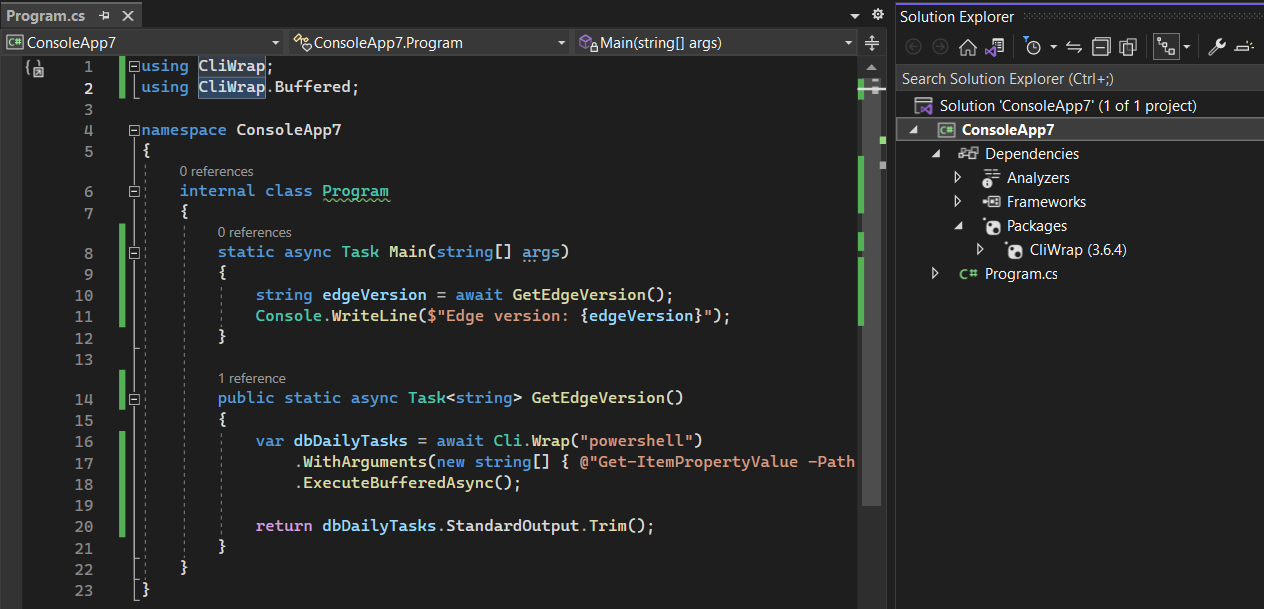
Conclusion
To get Edge version using C#, we can use RegistryKey
class to access the Windows Registry. Typically, the registry key resides under HKEY_CURRENT_USER\Software\Microsoft\Edge\BLBeacon
.
We can also get the information from installation location of Microsoft Edge. Then, in our C# code we construct a path to access msedge.exe
to retrieve the version using some codes related to FileVersionInfo
class.
Last, we can also use PowerShell script to get the edge version. We can use various packages to execute PowerShell script from C# such as CliWrap
or Microsoft.PowerShell.SDK
and System.Management.Automation
.