How to Get Default Browser from C#
Introduction
Knowing default browser information is important when we want to programmatically launching the browser and perform specific task that is enabled only by that browser.
In this article, we will show you how to get default browser information from C#.
Solution
Information about default browser can be retrieved from Windows Registry Key which is located at HKEY_CURRENT_USER\Software\Microsoft\Windows\Shell\Associations\UrlAssociations\http\UserChoice
. ProgId value will contain the browser name such as MSEdgeHTM
, IE.HTTP
, FirefoxURL
, ChromeHTML
, etc.
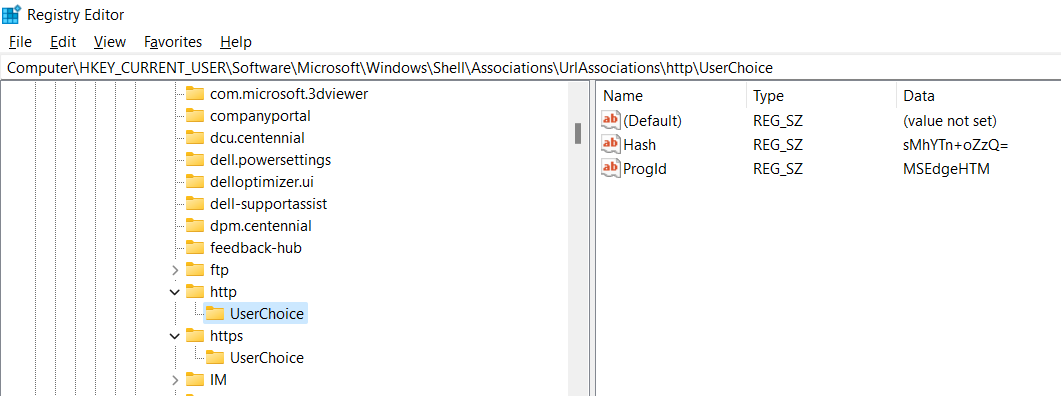
Using RegistryKey Class
To get information from Windows Registry, we can use RegistryKey
class from Microsoft.Win32
namespace. Then, we can use OpenSubKey
method to open each level of the keys.
using Microsoft.Win32;
using System.Diagnostics;
namespace ConsoleApp3
{
enum BrowserApplication
{
Edge,
InternetExplorer,
Firefox,
Chrome,
Opera,
Safari,
Unknown
}
internal class Program
{
static void Main(string[] args)
{
BrowserApplication defaultBrowser = GetDefaultBrowser();
Console.WriteLine("Default browser: " + defaultBrowser);
}
public static BrowserApplication GetDefaultBrowser()
{
BrowserApplication browser = BrowserApplication.Unknown;
try
{
using (RegistryKey userChoiceKey = Registry.CurrentUser.OpenSubKey(@"Software\Microsoft\Windows\Shell\Associations\UrlAssociations\http\UserChoice"))
{
if (userChoiceKey != null)
{
object progIdValue = userChoiceKey.GetValue("Progid");
if (progIdValue != null)
{
string progId = progIdValue.ToString();
switch (progId)
{
case "MSEdgeHTM":
browser = BrowserApplication.Edge;
break;
case "IE.HTTP":
browser = BrowserApplication.InternetExplorer;
break;
case "FirefoxURL":
browser = BrowserApplication.Firefox;
break;
case "ChromeHTML":
browser = BrowserApplication.Chrome;
break;
case "OperaStable":
browser = BrowserApplication.Opera;
break;
case "SafariHTML":
browser = BrowserApplication.Safari;
break;
default:
browser = BrowserApplication.Unknown;
break;
}
}
}
}
}
catch (Exception ex)
{
// Handle any exceptions
Debug.WriteLine("Error retrieving default browser: " + ex.Message);
}
return browser;
}
}
}
Using PowerShell Script
Since PowerShell is deeply integrated to .NET, we can execute PowerShell script through C#. In this case, we use CliWrap
package that enables us to work with any CLIs in C# like powershell
, cmd
, git
, npm
, docker
, etc.
In the following code, we create C# code that will execute PowerShell script (Get-ItemProperty HKCU:\Software\Microsoft\windows\Shell\Associations\UrlAssociations\http\UserChoice).Progid
behind the scene.
First, We need to add a reference to the CliWrap
package in our project and then add the code to call our PowerShell script.
using CliWrap;
using CliWrap.Buffered;
using System.Diagnostics;
namespace ConsoleApp4
{
enum BrowserApplication
{
Edge,
InternetExplorer,
Firefox,
Chrome,
Opera,
Safari,
Unknown
}
internal class Program
{
static async Task Main(string[] args)
{
BrowserApplication browser = await GetDefaultBrowser();
Console.WriteLine("Default browser: " + browser);
}
private static async Task<BrowserApplication> GetDefaultBrowser()
{
BrowserApplication browser = BrowserApplication.Unknown;
try
{
var dbDailyTasks = await Cli.Wrap("powershell")
.WithArguments(new string[]
{
@"(Get-ItemProperty HKCU:\Software\Microsoft\windows\Shell\Associations\UrlAssociations\http\UserChoice).Progid"
})
.ExecuteBufferedAsync();
string progId = dbDailyTasks.StandardOutput.Trim();
switch (progId)
{
case "MSEdgeHTM":
browser = BrowserApplication.Edge;
break;
case "IE.HTTP":
browser = BrowserApplication.InternetExplorer;
break;
case "FirefoxURL":
browser = BrowserApplication.Firefox;
break;
case "ChromeHTML":
browser = BrowserApplication.Chrome;
break;
case "OperaStable":
browser = BrowserApplication.Opera;
break;
case "SafariHTML":
browser = BrowserApplication.Safari;
break;
default:
browser = BrowserApplication.Unknown;
break;
}
}
catch (Exception ex)
{
// Handle any exceptions
Debug.WriteLine("Error retrieving default browser: " + ex.Message);
}
return browser;
}
}
}
Conclusion
Information about default browser is located at HKEY_CURRENT_USER\Software\Microsoft\Windows\Shell\Associations\UrlAssociations\http\UserChoice
. In C#, we can use RegistryKey
class from Microsoft.Win32
namespace.
Since, PowerShell is deeply integrated to .NET, hence C#, we can also get the information through PowerShell script executed from our C# code.